在 Week1 的学习中,我们已经知道 Many Time Pad 是不安全的,现在我们来动手破解它。
简要证明
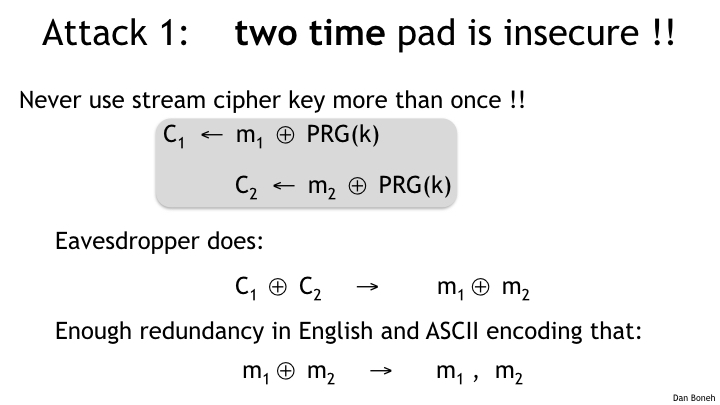
题目
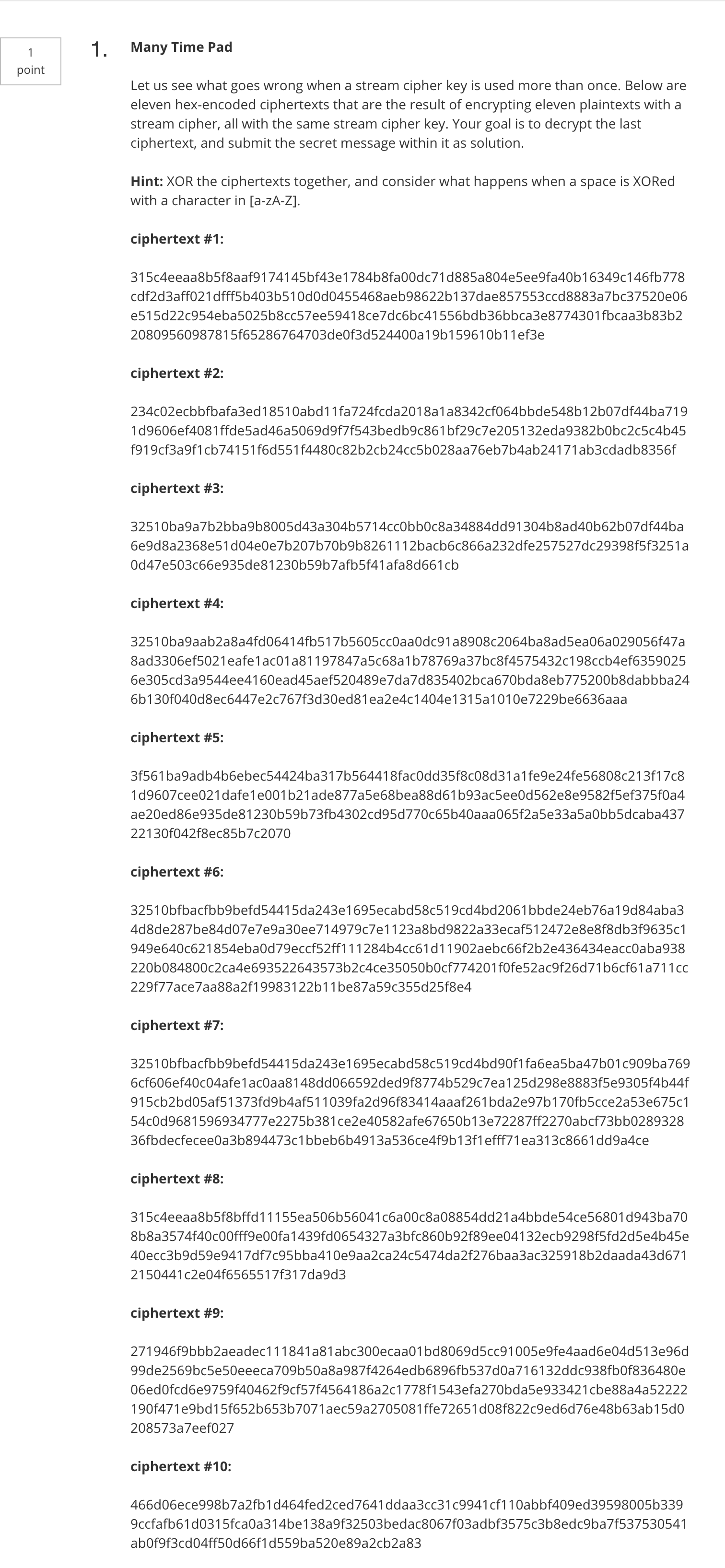
破解思路
我们首先定义合法字符集为{英文字母,[,], [.], [“], [ ]}
由于所有密文都是用同一密钥加密的,所以对于密文 $c_1$, $c_2$:
$$
\begin{align*}
result =& c_1 \oplus c_2 \oplus x (x 为合法字符)\\
result =& m_1 \oplus key \oplus m_2 \oplus key \oplus x (m_1, m_2, k 均为密文 c_1, c_2 以及密钥串 key 在同一位置的值)\\
\end{align*}
$$
排除意外碰撞,当且仅当 $m_1 \equiv x$ 或 $m_2 \equiv x$ 时,result 为合法字符
代码
1 | import binascii |
运行得到的值为:The sicuet cesiage is: Wrzn using a stream cipher, tever use the key more than onye
可以看到,并没有得到准确的原文,这里有两个因素:
- 合法字符集的范围可能不准确
- 我们并不能判断 $c_1 \equiv c_2 \equiv x$ 为 $m_1$ 还是 $m_2$
dic_list 中的每个 dict 都表示了相应位置每个合法字符的可能性,通过列出每个 dict 出现频次最高的 3 个字符,我们可以还原出原文:
The secret message is: when using a stream cipher, never use the key more than once